01 - Multiples of 3 or 5
Don’t say so much, just coding…
Instruction
If we list all the natural numbers below 10 that are multiples of 3 or 5, we get 3, 5, 6 and 9. The sum of these multiples is 23.
Finish the solution so that it returns the sum of all the multiples of 3 or 5 below the number passed in.
Note: If the number is a multiple of both 3 and 5, only count it once. Also, if a number is negative, return 0(for languages that do have them)
Ruby
Init
1 2 3
| def solution(number) end
|
Sample Testing
1 2 3 4 5 6 7 8 9
| def test(actual, expected) Test.assert_equals(actual, expected) end
Test.describe("example tests") do test(solution(10), 23) test(solution(20), 78) test(solution(200), 9168) end
|
Javascript
Init
1 2 3
| function solution(number){ }
|
Sample Testing
1 2 3 4 5 6 7 8
| function test(n, expected) { let actual = solution(n) Test.assertEquals(actual, expected, `Expected ${expected}, got ${actual}`) }
Test.describe("basic tests", function(){ test(10,23) })
|
Thinking
想法(1): 從題目概述 natural numbers below 10
中開始模擬會出現的數字
想法(2): 計算兩者的加總,可以知道在算 3 or 5 的倍數
想法(3): 處理級距,需要從 1 ~ 參數
然後再來選取符合項目,並加總
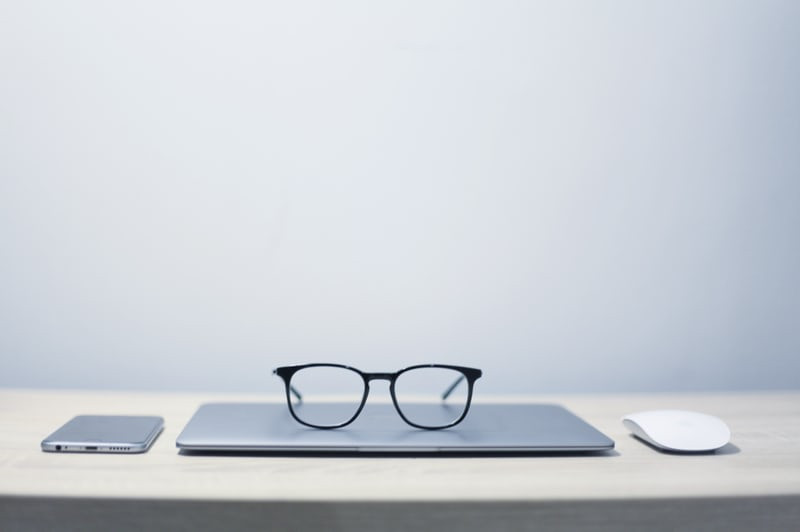
圖片來源:Unsplash Jesus Kiteque
Hint & Reference
Solution
Ruby
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| def solution(number) [*1..number-1].select{ |n| n % 3 == 0 || n % 5 == 0}.reduce(&:+) end
def solution(number) [*1...number].select{ |n| n % 3 == 0 || n % 5 == 0}.reduce(&:+) end
def solution(number) (1...number).select{ |n| n % 3 == 0 || n % 5 == 0}.inject(&:+) end
def solution(number) (1...number).select{ |n| (n % 5).zero? || (n % 3).zero?}.inject(:+) end
|
Javascript
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| function solution(number){ let sum = 0;
for(i = 1; i < number; i++){ if( i % 3 == 0 || i % 5 == 0){ sum += i; } } return sum; }
function solution(number){ return number < 3 ? 0 : [...Array(number).keys()] .map(int => (int % 3 === 0 || int % 5 === 0) ? int : 0 ) .reduce((a, b) => a + b ) }
|