06 - Character with longest consecutive repetition
Don’t say so much, just coding…
Instruction
For a given string s find the character c (or C) with longest consecutive repetition and return:
where l (or L) is the length of the repetition. If there are two or more characters with the same l return the first in order of appearance.
For empty string return:
Ruby
Init
1 2 3
| def longest_repetition chars end
|
Sample Testing
1 2 3 4 5 6 7 8 9 10 11 12
| describe "Solution" do it "should work for example tests" do Test.assert_equals(longest_repetition("aaaabb"), ['a', 4]) Test.assert_equals(longest_repetition("bbbaaabaaaa"), ['a', 4]) Test.assert_equals(longest_repetition("cbdeuuu900"), ['u', 3]) Test.assert_equals(longest_repetition("abbbbb"), ['b', 5]) Test.assert_equals(longest_repetition("aabb"), ['a', 2]) Test.assert_equals(longest_repetition("ba"), ['b', 1]) Test.assert_equals(longest_repetition(""), ['', 0]) Test.assert_equals(longest_repetition("aaabbcccddd"), ['a', 3]) end end
|
Javascript
Init
1 2 3
| function longestRepetition(s) { }
|
Sample Testing
1 2 3 4 5 6 7 8 9 10 11
| Test.describe("Longest repetition", ()=>{ Test.it("Example tests", function(){ Test.assertDeepEquals( longestRepetition("aaaabb"), ["a",4] ); Test.assertDeepEquals( longestRepetition("bbbaaabaaaa"), ["a",4] ); Test.assertDeepEquals( longestRepetition("cbdeuuu900"), ["u",3] ); Test.assertDeepEquals( longestRepetition("abbbbb"), ["b",5] ); Test.assertDeepEquals( longestRepetition("aabb"), ["a",2] ); Test.assertDeepEquals( longestRepetition(""), ["",0] ); Test.assertDeepEquals( longestRepetition("ba"), ["b",1] ); }); });
|
Thinking
想法(1): 與 見習村02 - Unique In Order 有點類似,都可以先將輸入的值分群
想法(2): 分群後的陣列,可以再來排序由大到小
想法(3): 排序完成後再來判斷如果傳進來的值是空的要另外回傳 ["", 0]
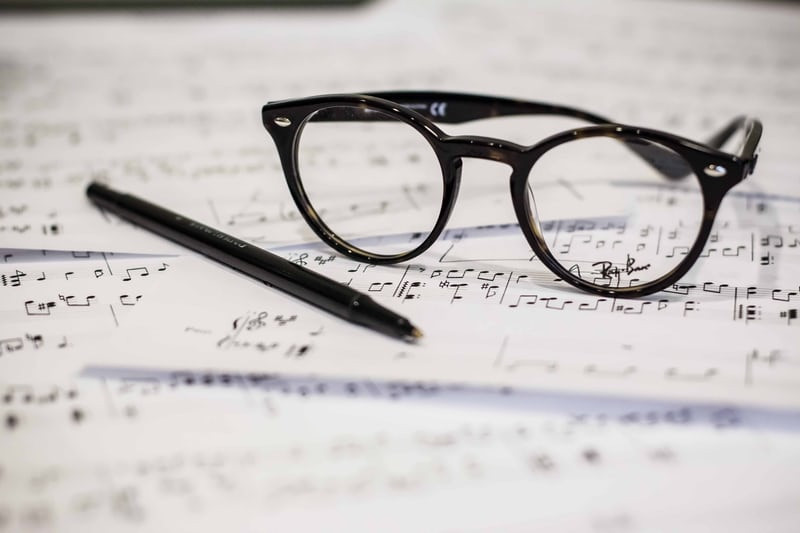
圖片來源:Unsplash Dayne Topkin
Hint & Reference
Solution
Ruby
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| def longest_repetition chars char = chars.gsub(/(.)\1*/).max_by(&:size) if char.nil? ['', 0] else [char.split('').first, char.split('').count] end end
def longest_repetition chars char = chars.gsub(/(.)\1*/).max_by(&:size) char.nil? ? ['', 0] : [char.split('').first, char.split('').count] end
def longest_repetition chars return ['', 0] if chars.empty? char = chars.gsub(/(.)\1*/).max_by(&:size).split('') [char.first, char.count] end
def longest_repetition chars return ["", 0] if chars.empty? chars.chars.chunk(&:itself) .map{ |char, chars| [char, chars.size] } .max_by { |char, size| size } end
|
Javascript
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| function longestRepetition(s) { let string = s.split(""); let result = ["", 0];
for(let i = 0; i < string.length; i++){ let repetitions = 1; while(string[i] == string[i+repetitions]){ repetitions++; } if(repetitions > result[1]){ result = [string[i],repetitions] } } return result; }
|