11 - Extract the domain name from a URL
Don’t say so much, just coding…
Instruction
Write a function that when given a URL as a string, parses out just the domain name and returns it as a string. For example:
1 2 3
| domain_name("http://github.com/carbonfive/raygun") == "github" domain_name("http://www.zombie-bites.com") == "zombie-bites" domain_name("https://www.cnet.com") == "cnet"
|
Ruby
Init
1 2 3
| def domain_name(url) end
|
Sample Testing
1 2 3 4
| Test.assert_equals(domain_name("http://google.com"), "google") Test.assert_equals(domain_name("http://google.co.jp"), "google") Test.assert_equals(domain_name("www.xakep.ru"), "xakep") Test.assert_equals(domain_name("https://youtube.com"), "youtube")
|
Javascript
Init
1 2 3
| function domainName(url){ }
|
Sample Testing
1 2 3 4
| Test.assertEquals(domainName("http://google.com"), "google"); Test.assertEquals(domainName("http://google.co.jp"), "google"); Test.assertEquals(domainName("www.xakep.ru"), "xakep"); Test.assertEquals(domainName("https://youtube.com"), "youtube");
|
Thinking
想法(1): 原本其實是從 URI
的方式寫完的,不過發現要處理的東西有點多,後來覺得 start_with
來解決就可以,也不用 regex
去 metch 所有條件(regex 就爛)
想法(2): 用 gsub
把所有要篩選的條件寫進來也不錯
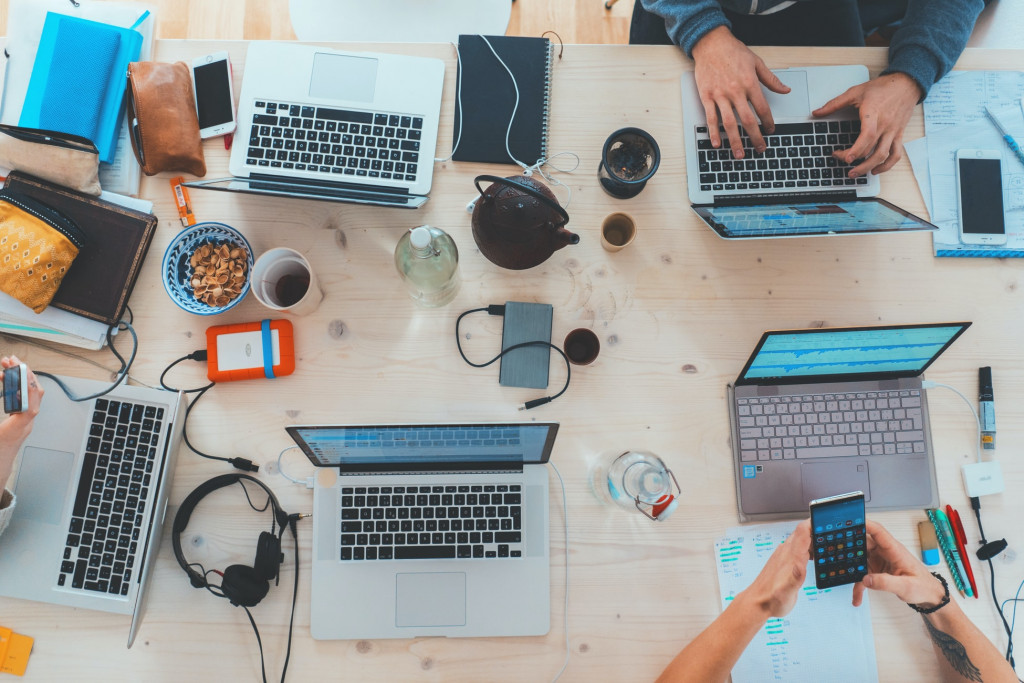
圖片來源:Unsplash Marvin Meyer
Hint & Reference
Solution
Ruby
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| def domain_name(url) url = url.start_with?('www.') ? url.split('www.').last : url.split('//').last url.split('.').first end
def domain_name(url) url.start_with?('www.') ? url.split('www.').last.split('.').first : url.split('//').last.split('.').first end
def domain_name(url) url.gsub('http://', '') .gsub('https://', '') .gsub('www.', '') .split(".")[0] end
|
Javascript
1 2 3 4 5 6 7
| function domainName(url){ return url.replace('http://', '') .replace('https://', '') .replace('www.', '') .split('.')[0]; }
|