20 - Scramblies
Don’t say so much, just coding…
Instruction
Complete the function scramble(str1, str2) that returns true if a portion of str1 characters can be rearranged to match str2, otherwise returns false.
Notes:
- Only lower case letters will be used (a-z). No punctuation or digits will be included.
- Performance needs to be considered
1
| Input strings s1 and s2 are null terminated.
|
Examples
1 2 3
| scramble('rkqodlw', 'world') ==> True scramble('cedewaraaossoqqyt', 'codewars') ==> True scramble('katas', 'steak') ==> False
|
Ruby
Init
1 2 3
| def scramble(s1,s2) end
|
Sample Testing
1 2 3 4 5
| Test.assert_equals(scramble('rkqodlw','world'),true) Test.assert_equals(scramble('cedewaraaossoqqyt','codewars'),true) Test.assert_equals(scramble('katas','steak'),false) Test.assert_equals(scramble('scriptjava','javascript'),true) Test.assert_equals(scramble('scriptingjava','javascript'),true)
|
Javascript
Init
1 2 3
| function scramble(str1, str2) { }
|
Sample Testing
1 2 3 4 5 6 7 8 9 10
| describe('Example Tests', function(){ Test.assertEquals(scramble('rkqodlw','world'),true); Test.assertEquals(scramble('cedewaraaossoqqyt','codewars'),true); Test.assertEquals(scramble('katas','steak'),false); Test.assertEquals(scramble('scriptjava','javascript'),true); Test.assertEquals(scramble('scriptingjava','javascript'),true); Test.assertEquals(scramble('scriptsjava','javascripts'),true); Test.assertEquals(scramble('jscripts','javascript'),false); Test.assertEquals(scramble('aabbcamaomsccdd','commas'),true); });
|
Thinking
想法(1): 後者的值,跟前者比較時,要所有的字都有在前者且數量也要ㄧ樣
想法(2): 將後者的值透過 uniq
後,跑迴圈來比較 count
有無 小於等於
前者
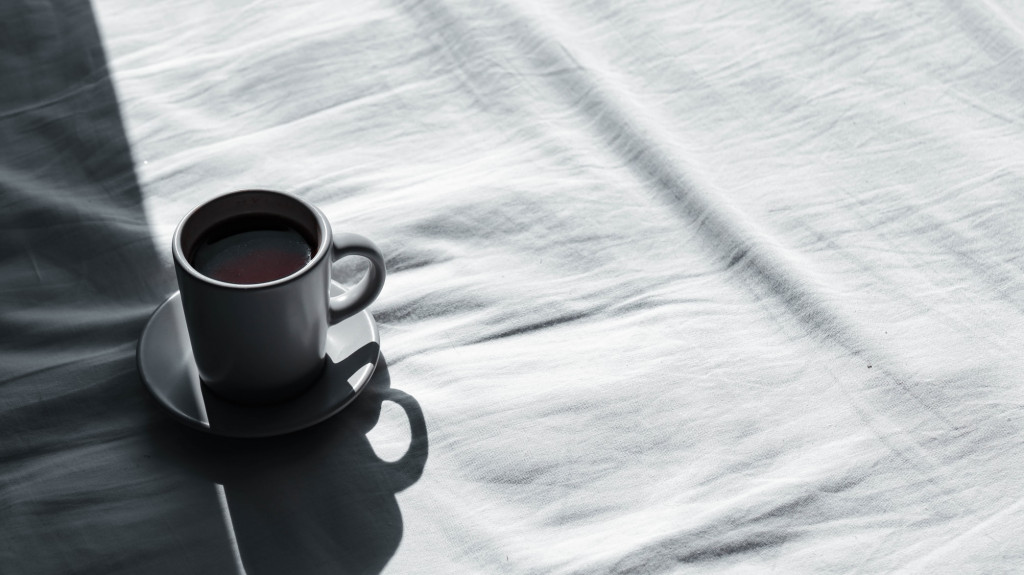
圖片來源:Unsplash Buse Doga Ay
Hint & Reference
Solution
Ruby
1 2 3 4 5 6 7 8 9 10 11 12 13
| def scramble(s1,s2) s3 = s1.split("") s2.split("").each do |x| s3.each_with_index{ |y,i| (s3.delete_at i ; break) if y == x } end s1.length - s2.length == s3.join.length ? true : false end
def scramble(s1,s2) s2.chars.uniq.all?{ |x| s2.count(x) <= s1.count(x) } end
|
Javascript
1 2 3 4
| function scramble(str1, str2) { return [...str2].every(val => str2.split(val).length <= str1.split(val).length); }
|