30 - A Chain adding function
Don’t say so much, just coding…
Instruction
We want to create a function that will add numbers together when called in succession.
We also want to be able to continue to add numbers to our chain.
1 2 3
| add(1)(2)(3); add(1)(2)(3)(4); add(1)(2)(3)(4)(5);
|
and so on.
A single call should return the number passed in.
We should be able to store the returned values and reuse them.
1 2 3 4 5
| var addTwo = add(2); addTwo; addTwo + 5; addTwo(3); addTwo(3)(5);
|
We can assume any number being passed in will be valid whole number.
Ruby
Init
Sample Testing
1 2 3
| Test.expect(add(1) == 1); Test.expect(add(1).(2) == 3); Test.expect(add(1).(2).(3) == 6);
|
Javascript
Init
Sample Testing
1 2 3
| Test.expect(add(1) == 1); Test.expect(add(1)(2) == 3); Test.expect(add(1)(2)(3) == 6);
|
Thinking
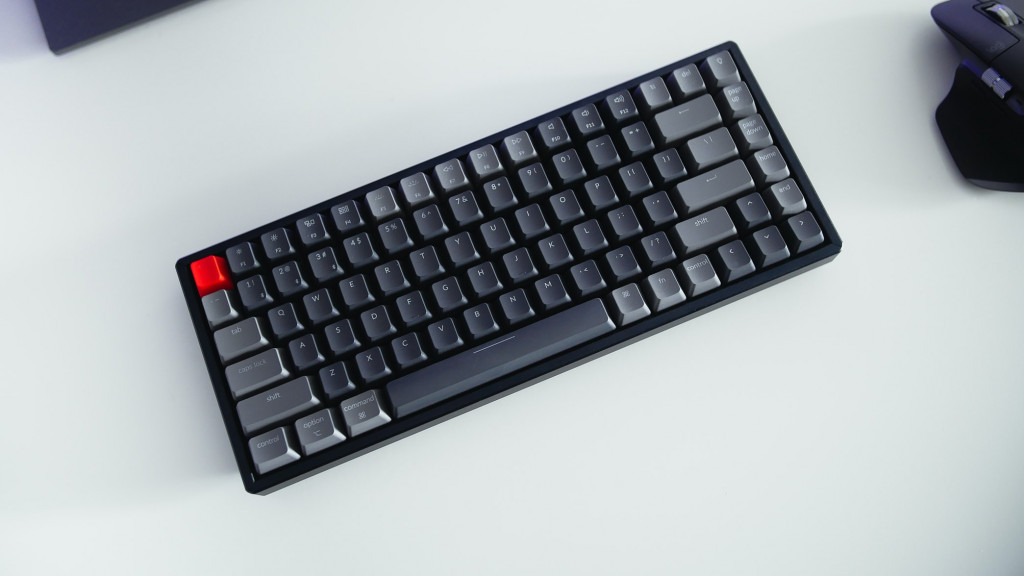
圖片來源:Unsplash Martin Garrido
Hint & Reference
Solution
Ruby
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| def add(n) n end
class Fixnum def call(m) self + m end end
def add(n) def call(m) return self + m end return n end
|
Javascript
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| function add(n){ var fn = function(x) { return add(n + x); }; fn.valueOf = function() { return n; }; return fn; }
function add(n) { const f = x => add(n + x) f.valueOf = () => n return f; }
|